This cheat sheet sums up the basics of C#, for experienced developers who are learning C# and users who already know programming basics, hopefully this document has helped you in some way, there was not much information or explaining but then again I’m assuming you’ve. Find color c, the average between colors c1, c2. C, c1, c2 are strings of hex color codes: 7 chars, beginning with a number sign #. Assume linear computations, ignore gamma corrections. Using System.Drawing. In C#, a list is a generic data structure that can hold any type. Use the new operator and declare the element type in the angle brackets. In the example code, names is a list containing string values.someObjects is a list containing Object instances.
The XML documentation tags of C# are described very well in the MSDN. However, the article does not explain how the contained phrases and sentences should be written. This article tries to fill this gap by providing rules and some sample phrases.

I recommend using StyleCop because its rules enforce some of the XML documentation recommendations from this article. Also check out the Visual Studio extension GhostDoc which automates and simplifies the writing of XML documentation.
General
All XML documentation phrases should end with a period (.) and no blank:
The
summary
tags should only contain the most important information. For further details use an additionalremarks
tag. To avoid having too much documentation in your source code files, read this article which explains how to “outsource” documentation to an external file.
Classes
Each class should have a
summary
tag describing its responsibility. The summary often starts with “Represents …“ or “Provides …“ but other forms also exist:
Constructors
The documentation of a constructor should be in the form “Initializes a new instance of the <see cref=”TYPENAME”/> class.”</see>:
Properties
The summary of a property should start with “Gets or sets …“ if it is fully accessible, with “Gets …“ when the property is read-only, and with “Sets …“ if it is write-only:
Note: According to the very recommended book Framework Design Guidelines, write-only properties should not be used at all.
A property of type
bool
should start with “Gets or sets a value indicating whether …“:
Events
Each event should have a
summary
tag which starts with the words “Occurs when …“:Note: Events should be named with a verb or verb phrase. Use the present and past tenses to express if the delegate is invoked before (e.g.
Closing
) or after (e.g.Closed
) a particular event.
Methods
Like the method name itself, the text in the
summary
tag should start with a verb:
Method parameters
Each method parameter should have a corresponding
param
tag containing a description of the parameter:If the parameter is an
enum
or of typebool
consider starting the description with “Specifies …“ (enum) or “Specifies whether …“ (bool)Note: According to Clean Code you should not use
bool
method parameters.
Method return value
If the method returns a value, you should add a
returns
tag which contains a description of the returned object:If the returned value is of type
bool
the documentation should be in the form “true if CONDITION; otherwise,false .”:
Exceptions
The exception
exception
tag contains the reason why the exception occurred:Check designer download. Tip: Have a look at Exceptional for ReSharper which greatly helps creating XML documentation for exceptions.
Final words
The rules in this articles are used in StyleCop and in the .NET Framework, therefore I think using them is best practice. Because it looks more clean to me, I write the opening tag, content and closing tag on a single line. Of course you can write them on multiple lines as proposed by the Visual Studio templates. For the generated XML documentation file it does not matter what style you are using.
What do you think about the described XML documentation rules? Are some rules missing in this list?
Rico Suter SOFTWARE ENGINEERING EDIT
Best PracticesXML Documentation.NETC#Cheat SheetClean CodeCoding GuidelinesDocumentation
Introduction
C# is an objected oriented language that runs on Microsoft’s .NET Framework, C# can as well be run on other frameworks (E.g. Unity)
.NET is an open source platform to develop application
Set up dotnet core
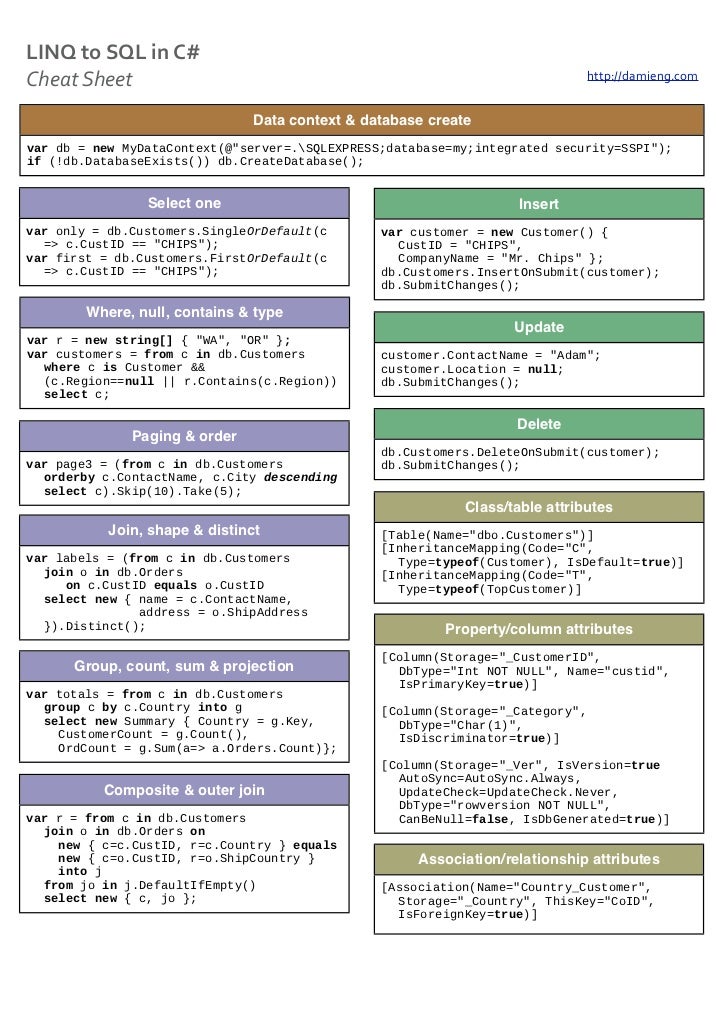
Install
In this article, I will use .NET core 2.2 but you can use the latest version if available. .NET core can be run on Windows, Linux and macOS.
You can install the dotnet core SDK from Microsoft
Once .NET core is installed you can run this one using the CLI
Run:
Output:
Create an app
Create a new project called myConsolApp. Open the folder in your terminal and run:
.NET core will create a basic program in the myConsolApp folder. You should have the two following files:
- Program.cs: This file contains the basic default program, that will print “Hello world!”
- myConsolApp.csproj: This file has the information needed to build and run our program
Program.cs:
- Namespace: declare a scope that contains a set of related objects | Microsoft - docs
- Class: In object oriented programming, a class is a blueprint / template for data types. It encapsulates variables, methods and other types. | Microsoft - docs
- Method: A method is a code block that contains a series of statements.
static void
means that theMain
method is not a return method | Microsoft - docs
Let’s try to run our program:
Output:

When running dotnet run
, by default .NET will look for the method Main()
. This Main()
method is the entry point of our console application. If we rename this one, the program will break.
Run:
Output:
Let’s revert this last change 😬.
We have now a basic project where we are going to be able to experiment with C#.
In C# you can declare 2 kinds of comments:
Variables, constants and data types
Types

C# is a strongly-typed language. Every variable and constant has a type.
When declaring a variable we need to set its type:
When performing an operation with variables of different types, you need to be careful of their types, or you might get unexpected behaviour.
Here are the default value types available by default in C#
Type | Represents | Range |
---|---|---|
bool | Boolean value | True or False |
byte | 8-bit unsigned integer | 0 to 255 |
char | 16-bit Unicode character | U +0000 to U +ffff |
decimal | 128-bit precise decimal values with 28-29 significant digits | (-7.9 x 1028 to 7.9 x 1028) / 100to 28 |
double | 64-bit double-precision floating point type | (+/-)5.0 x 10-324 to (+/-)1.7 x 10308 |
float | 32-bit single-precision floating point type | -3.4 x 1038 to + 3.4 x 1038 |
int | 32-bit signed integer type | -2,147,483,648 to 2,147,483,647 |
long | 64-bit signed integer type | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
sbyte | 8-bit signed integer type | -128 to 127 |
short | 16-bit signed integer type | -32,768 to 32,767 |
string | Sequence of zero or more Unicode characters | |
uint | 32-bit unsigned integer type | 0 to 4,294,967,295 |
ulong | 64-bit unsigned integer type | 0 to 18,446,744,073,709,551,615 |
ushort | 16-bit unsigned integer type | 0 to 65,535 |
var
keyword
Variables of anonymous types can be declared using the var
keyboard.
const
keyword
const
is used de declare a constant, it can’t be modified.
Arrays
An array can store multiple variables of the same type. An array has a fixed size.
type[] myArray
Single dimensional arrays
You can access the value of an array item using its index:
Multidimensional arrays
Array can have multiple dimensions, each array in the multidimensional arrays have the same length.
Jagged Arrays
Bmw e39 rear door wont open. Jagged arrays are similar to multidimensional ones, but all elements of this array can have different dimensions and sizes.
Lists
Namespace:System.Collections.Generic
A list represents a strongly typed list of objects that can be accessed by index. It is less performant than an array. It doesn’t have a fixed size.
List<type> myList
Methods
A method refers to a block of code, It executes a series of instruction.
A method in C# can be declared in a class
or a struct
. Methods follow the following structure:
- Static modifier is optional, a static method or variable is not associated with the instance (class or struct).
- Access Specifier specifies how the method can be accessed from another class
- public: No access restrictions, the method can be accessed from other classes.
- private: Access restricted, the method can be accessed only from the class it has been declared in.
- Return Type, methods can return a value, if it is the case you need to specify the data type that the method will return (E.g.
string[]
,int
,bool
…) and you will need areturn
keyword in the method body. If the method does not return a value, then you can use thevoid
keyword. - Method Name: Unique method name.
- Parameters, pass data to the method.
- Method Body contains the set of instructions needed if it is a return method you will need to
return
a value.
Method example 1 - private void
Method example 2 - static private void
Method example 3 - static public string
Statements examples
C# has different statements available:
if else
switch
for
foreach
do
The do statements get executed at least once, as the expression is evaluated after each execution of the loop.
C# Programming Cheat Sheet
while
C Sharp Cheat Sheets
Resources
